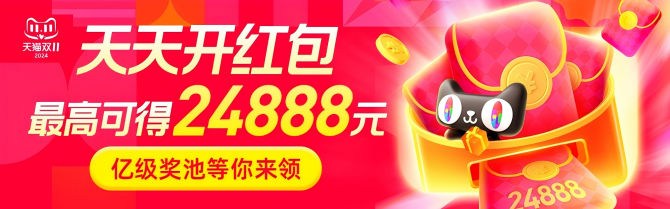
linux命令模式下如何看pdf文件?
linux命令模式下查看pdf文件需要借助evince命令,如打开当前目录下的a.pdf文件的命令是evince a.pdf注意:在Linux的文本模式下是不能使用该命令并查看pdf文件的。可以实施fbgs,但只能查看部分pdf文档。说明:Evince 原本是 GNOME 环境中一个简单的文档查看器,可以查看 PDF、Postscript、djvu、tiff、dvi 等文档。
java如何实现在web工程中用OpenOffice生成带有图片水印的pdf?
需要itext2.1.5,
以下是对pdf加水印的代码,包括文字水印和图片水印
public int fileCopy(String srcPath, String destPath) {
FileOutputStream fos = null;
FileInputStream fis = null;
try {
fos = new FileOutputStream(destPath);
fis = new FileInputStream(srcPath);
byte buffer = new byte;
int len = 0;
while ((len = fis.read(buffer)) > 0) {
fos.write(buffer, 0, len);
}
return 1;
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
fis.close();
fos.flush();
fos.close();} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
return 0;
}/**
* 为pdf文件加文字水印
*
* @param srcPath
* 源文件路径
* @param destPath
* 目标文件路径
* @param waterText
* 水印文字
* @throws DocumentException
* @throws IOException
*/
public void wordWaterMark(String srcPath, String destPath, String waterText) throws DocumentException, IOException {
int result = fileCopy(srcPath, destPath);
if (result == 1) {
// 待加水印的文件
PdfReader reader = new PdfReader(destPath);
// 加完水印的文件
PdfStamper stamper = new PdfStamper(reader, new FileOutputStream(srcPath));int total = reader.getNumberOfPages() + 1;
PdfContentByte content;
// 设置字体
BaseFont base = BaseFont.createFont(fontPath, BaseFont.IDENTITY_H, BaseFont.NOT_EMBEDDED);// 水印文字
int j = waterText.length(); // 文字长度
char c = 0;
int high = 0;// 高度
// 循环对每页插入水印
for (int i = 1; i < total; i++) {
// 水印的起始
high = 60;
content = stamper.getUnderContent(i);
PdfGState gs = new PdfGState();
gs.setFillOpacity(0.1f);// 设置透明度为0.2
content.setGState(gs);
// 开始
content.beginText();
// 设置颜色
// content.setColorFill(new Color());
// 设置字体及字号
content.setFontAndSize(base, 88);
// 设置起始位置
content.setTextMatrix(120, 333);
// 开始写入水印
for (int k = 0; k < j; k++) {
content.setTextRise(high);
c = waterText.charAt(k);
content.showText(c + “”);
high += 20;
}
content.endText();}
stamper.close();
System.out.println(“添加成功++++++++++++++++++++++++++++++++++++++++++”);
} else {
System.out.println(“复制pdf失败====================”);
}}
public void picWaterMark(String srcPath, String destPath, String imageFilePath)
throws DocumentException, IOException {
int result = fileCopy(srcPath, destPath);
if (result == 1) {
// 待加水印的文件
PdfReader reader = new PdfReader(destPath);
// 加完水印的文件
PdfStamper stamper = new PdfStamper(reader, new FileOutputStream(srcPath));
Image img = Image.getInstance(imageFilePath);
img.setAbsolutePosition(50, 400);// 坐标
img.setRotation(20);// 旋转 弧度
img.setRotationDegrees(45);// 旋转 角度
// image.scaleAbsolute(200,100);//自定义大小
img.scalePercent(50);// 依照比例缩放
int pageSize = reader.getNumberOfPages();
for (int i = 1; i <= pageSize; i++) {
PdfContentByte under = stamper.getUnderContent(i);
under.addImage(img);
PdfGState gs = new PdfGState();
gs.setFillOpacity(0.2f);// 设置透明度为0.2
under.setGState(gs);
}
stamper.close();// 关闭
System.out.println(“添加成功++++++++++++++++++++++++++++++++++++++++++”);
} else {
System.out.println(“复制pdf失败====================”);
}
}linux下转pdf可以用libreoffice,需要安装,这个是免费的,具体代码如下:
String command = “libreoffice5.0 –invisible –convert-to pdf:writer_pdf_Export –outdir ” + destFilepath + ” ” + source; try { p = Runtime.getRuntime().exec(command); p.waitFor(); } catch (InterruptedException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); }