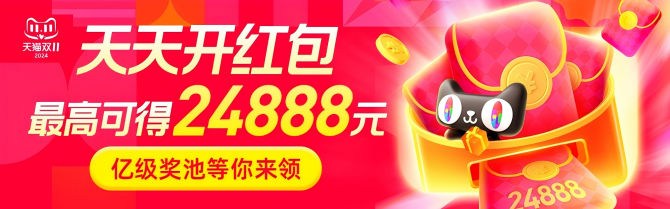
一、SQLite3 数据库的安装
1、安装
(1)本地安装
sudo dpkg -i *.deb
sudo —–以管理员身份执行
dpkg —–软件包管理器
-i —–安装
*.deb —–所有deb软件包
(2)在线安装
sudo apt-get install sqlite3
二、SQLite 基本命令
1、系统命令
以‘.’
开头的命令
.help ----帮助
.exit ----退出
.quit ----退出
.schema ----查看表的结构图
2、sql命令
(1)创建一张数据表 stu
表名(字段名 字段类型)
create table stu(id Integer, name char, score Integer);
(2)插入一条记录
insert into stu values(1001, 'zhangsan', 80);
插入部分字段的操作:
insert into stu(id, name) values(1001, 'zhangsan');
(3)查询记录
select * form stu;
查询部分字段:
select name,score from stu,
按指定条件查询:
select * from stu where score=80 and id=1001;
(4)删除记录
delete from stu where id=1;
(5)更新/修改记录
update stu set name=‘王五’ where id=100;
update stu set name=‘王五’,score=88 where id=100;
三、SQLite 进阶命令
1、系统命令
.database ----查看打开数据库的路径
.table ----查看表
2、sql命令
(1)插入一列
alter table stu add column address char;
用 .schema
查看下表结构,可以看到多了一列
(2)删除一列
SQLite3不支持,直接删除一列
1)创建一张表,从旧表种提取字段
2)删除原有的表
3)将新的表名字改成原有的旧的表的名字
create table tmp as select id, name, score from stu;
drop table stu;
alter table tmp rename to stu;
四、SQLite3 编程接口
1、基本接口
https://www.sqlite.org/c3ref/funclist.html
int sqlite3_open(const char *filename, /* Database filename (UTF-8) */sqlite3 **ppDb /* OUT: SQLite db handle */
);
功能:打开数据库
参数: ppDb 数据库的操作句柄(指针)
返回值:成功SQLITE_OK,失败错误码
int sqlite3_close(sqlite3*);
const char *sqlite3_errmsg(sqlite3*);
功能:打印错误信息
返回值:错误信息的首地址
int sqlite3_exec(sqlite3*, /* An open database */const char *sql, /* SQL to be evaluated */int (*callback)(void*,int,char**,char**), /* Callback function */void *, /* 1st argument to callback */char **errmsg /* Error msg written here */
);
功能:执行一条sql语句
参数:sql 一条sql语句callback 回调函数,每找到一条记录自动执行一次回调函数errmsg 错误消息回调函数的功能:查询的结果,只有sql为查询语句的时候,才会执行此语句
回调函数的参数:void* 传递给回调函数的参数int 记录包含的字段数目char** 包含每个字段值的指针数组char** 包含每个字段名称的指针数组
回调函数的返回值: 成功返回0;失败返回-1
sqlite3_free(errmsg); 释放错误信息
if(errmsg != NULL)
{
sqlite3_free(errmsg); //这里需要用sqlite3_free去free,切忌!
}
3、不使用回调函数进行查询
int sqlite3_get_table(sqlite3 *db, /* An open database */句柄const char *zSql, /* SQL to be evaluated */char ***pazResult, /* Results of the query */查询结果int *pnRow, /* Number of result rows written here */行数int *pnColumn, /* Number of result columns written here */列数char **pzErrmsg /* Error msg written here */错误信息
);
void sqlite3_free_table(char **result);