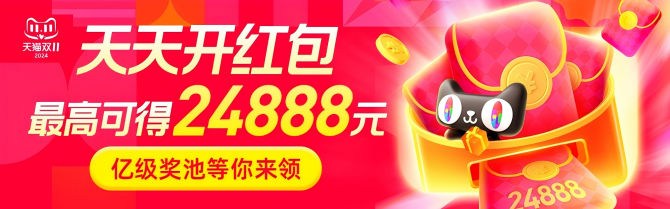
python函数参数定义
Python函数定义中的5种参数类型: (5 Types of Arguments in Python Function Definition:)
-
default arguments
default arguments
-
keyword arguments
keyword arguments
-
positional arguments
positional arguments
-
arbitrary positional arguments
arbitrary positional arguments
-
arbitrary keyword arguments
arbitrary keyword arguments
Python函数定义: (Python Function Definition:)
The function definition starts with the keyword def
. It must be followed by the function name and the parenthesized list of formal parameters. The statements that form the body of the function start at the next line and must be indented.
函数定义以关键字def
开头。 它后面必须是函数名称和形式参数的括号列表。 构成函数主体的语句从下一行开始,并且必须缩进。

Formal parameters are mentioned in function definition .Actual parameters(arguments) are passed during function call.
函数定义中提到了形式参数 。 实际参数(参数)在函数调用期间传递。
We can define a function with a variable number of arguments
我们可以定义一个带有可变数量参数的函数
1.默认参数 (1.default arguments:)
- default arguments are values that are provided while defining functions.
默认参数是定义函数时提供的值。
-
The assignment operator
=
is used to assign a default value to the argument.赋值运算符
=
用于为参数分配默认值。 - default arguments become optional during the function calls.
默认参数在函数调用期间变为可选。
- If we provide value to the default arguments during function calls, it overrides the default value.
如果我们在函数调用期间为默认参数提供值,它将覆盖默认值。
- The function can have any number of default arguments
该函数可以具有任意数量的默认参数
- Default arguments should be followed by non-default arguments.
默认参数后应跟非默认参数。
Example:
例:
In the below example, the default value is given to argument b
,c
在下面的示例中,默认值被赋予参数b
, c
def add(a,b=5,c=10):return (a+b+c)
This function can be called in 3 ways
可以通过3种方式调用此函数
-
Giving only the mandatory argument
仅给出强制性参数
print(add(3))#Output:18
2. Giving one of the optional arguments.3 is assigned to a, 4 is assigned to b.
2.提供一个可选参数。 3分配给a,4分配给b。
print(add(3,4))#Output:14
3.Giving all the arguments
3,给出所有的论点
print(add(2,3,4))#Output:9
Note: Default values are evaluated only once at the point of the function definition in the defining scope. So, it makes a difference when we pass mutable objects like list,dictionary as default values.
注意:默认值在定义范围中的函数定义点仅被评估一次。 因此,当我们将可变对象(如list,dictionary)作为默认值传递时,它会有所不同。
2.关键字参数: (2. Keyword Arguments:)
Functions can also be called using keyword arguments of the form kwarg=value.
也可以使用kwarg=value.
形式的关键字参数来调用函数kwarg=value.
During function call, values passed through arguments need not be in the order of parameters in the function definition. This can be achieved by keyword arguments. But all the keyword arguments should match the parameters in the function definition.
在函数调用期间,通过参数传递的值不必按函数定义中参数的顺序。 这可以通过关键字参数来实现。 但是所有关键字参数都应与函数定义中的参数匹配。
Example:
例:
def add(a,b=5,c=10):return (a+b+c)
Calling the function add
by giving keyword arguments
通过给关键字参数调用function add
- All parameters are given as keyword arguments. So no need to maintain the same order.
所有参数均作为关键字参数给出。 因此,无需维护相同的顺序。
print (add(b=10,c=15,a=20))#Output:45
2. During function call, only giving mandatory argument as a keyword argument. Optional default arguments are skipped.
2.在函数调用期间,仅提供强制性参数作为关键字参数。 跳过可选的默认参数。
print (add(a=10))#Output:25
3.位置参数 (3. Positional Arguments)
During function call, values passed through arguments should be in the order of parameters in the function definition. This is called positional arguments.
在函数调用期间,通过参数传递的值应按函数定义中参数的顺序排列。 这称为位置参数。
Keyword arguments should follow positional arguments only.
关键字参数应仅跟随位置参数。
Example:
例:
def add(a,b,c):return (a+b+c)
The above function can be called in two ways:
可以通过两种方式调用以上函数:
-
During function call, all arguments are given as positional arguments. Values passed through arguments are passed to parameters by their position.
10
is assigned toa
,20
is assigned tob
and30
is assigned toc
在函数调用期间,所有参数都作为位置参数给出。 通过参数传递的值按其位置传递给参数。
10
分配给a
,20
分配给b
,30
分配给c
print (add(10,20,30))#Output:60
2. Giving a mix of positional and keyword arguments. keyword arguments should always follow positional arguments
2.混合使用位置参数和关键字参数。 关键字参数应始终跟随位置参数
print (add(10,c=30,b=20))#Output:60
Default vs positional vs keyword arguments:
默认vs位置vs关键字参数:

Important points to remember:
要记住的要点:

1.default arguments should follow non-default arguments
1.默认参数应遵循非默认参数
def add(a=5,b,c):return (a+b+c)#Output:SyntaxError: non-default argument follows default argument
2. keyword arguments should follow positional arguments
2.关键字参数应跟随位置参数
def add(a,b,c):return (a+b+c)
print (add(a=10,3,4))#Output:SyntaxError: positional argument follows keyword argument
3. All the keyword arguments passed must match one of the arguments accepted by the function and their order is not important.
3.传递的所有关键字参数必须与函数接受的参数之一匹配,并且它们的顺序并不重要。
def add(a,b,c):return (a+b+c)
print (add(a=10,b1=5,c=12))#Output:TypeError: add() got an unexpected keyword argument 'b1'
4.No argument should receive a value more than once
4,任何参数都不应多次收到一个值
def add(a,b,c):return (a+b+c)
print (add(a=10,b=5,b=10,c=12))#Output:SyntaxError: keyword argument repeated
5. Default arguments are optional arguments
5.默认参数是可选参数
Example 1: Giving only the mandatory arguments
示例1:仅提供强制参数
def add(a,b=5,c=10):return (a+b+c)
print (add(2))#Output:17
Example 2: Giving all arguments(optional and mandatory arguments)
示例2:给出所有参数(可选和强制参数)
def add(a,b=5,c=10):return (a+b+c)
print (add(2,3,4))#Output:9
变长参数 (Variable-length arguments)
Variable-length arguments are also known as arbitary arguments. If we don’t know the number of arguments needed for the function in advance, we can use arbitary arguments
可变长度自变量也称为任意变量 。 如果我们事先不知道函数所需的参数数量,则可以使用任意参数
Two types of arbitrary arguments
两种类型的任意参数
- arbitary positional arguments
任意位置论证
- arbitrary keyword arguments
任意关键字参数
4.任意位置参数: (4. arbitrary positional arguments:)
For arbitrary positional argument, an asterisk (*) is placed before a parameter in function definition which can hold non-keyword variable-length arguments. These arguments will be wrapped up in a tuple. Before the variable number of arguments, zero or more normal arguments may occur.
对于任意位置参数,在函数定义中的参数之前放置星号(*) ,该参数可以容纳非关键字可变长度参数。 这些论点将包裹在一个元组中 。 在可变数量的参数之前,可能会出现零个或多个常规参数。
def add(*b):
result=0for i in b:
result=result+ireturn result
print (add(1,2,3,4,5))#Output:15print (add(10,20))#Output:30
5,任意关键字参数 (5.arbitrary keyword arguments:)
For arbitrary positional argument, a double asterisk (**) is placed before a parameter in function which can hold keyword variable-length arguments.
对于任意位置参数,函数中的参数前可以放置一个双星号(**) ,该参数可以容纳关键字可变长度参数。
Example:
例:
def fn(**a):for i in a.items():
print (i)
fn(numbers=5,colors="blue",fruits="apple")'''
Output:
('numbers', 5)
('colors', 'blue')
('fruits', 'apple')
'''
特殊参数: (Special Parameters:)
As per Python Documentation
根据Python文档
By default, arguments may be passed to a Python function either by position or explicitly by keyword. For readability and performance, it makes sense to restrict the way arguments can be passed so that a developer need only look at the function definition to determine if items are passed by position, by position or keyword, or by keyword.
默认情况下,参数可以按位置或通过关键字显式传递给Python函数。 为了提高可读性和性能,限制参数传递的方式是有意义的,以便开发人员仅需查看函数定义即可确定是按位置,按位置或关键字还是按关键字传递项目。
A function definition may look like:
函数定义可能类似于:

where /
and *
are optional. If used, these symbols indicate the kind of parameter by how the arguments may be passed to the function: positional-only, positional-or-keyword, and keyword-only.
/
和*
是可选的。 如果使用这些符号,则它们通过将参数传递给函数的方式指示参数的类型:仅位置,仅位置关键字或仅关键字。
- Positional or keyword arguments
位置或关键字参数
- Positional only parameters
仅位置参数
- keyword-only arguments
仅关键字参数
1,位置或关键字参数 (1.Positional or keyword arguments)
If /
and *
are not present in the function definition, arguments may be passed to a function by position or by keyword
如果函数定义中不存在/
和*
,则可以按位置或关键字将参数传递给函数
def add(a,b,c):return a+b+c
print (add(3,4,5))#Output:12print (add(3,c=1,b=2))#Output:6
2. Positional only parameters
2.仅位置参数
Positional-only parameters are placed before a /
(forward-slash)in the function definition. The /
is used to logically separate the positional-only parameters from the rest of the parameters. Parameters following the /
may be positional-or-keyword or keyword-only.
仅位置参数位于函数定义中的/
(正斜杠)之前。 /
用于在逻辑上将仅位置参数与其余参数分开。 /
之后的参数可以是positionor-or-keyword或only -keyword 。
def add(a,b,/,c,d):return a+b+c+d
print (add(3,4,5,6))#Output:12print (add(3,4,c=1,d=2))#Output:6
If we specify keyword arguments for positional only arguments, it will raise TypeError.
如果我们为仅位置参数指定关键字参数,则会引发TypeError。
def add(a,b,/,c,d):return a+b+c+d
print (add(3,b=4,c=1,d=2))#Output:TypeError: add() got some positional-only arguments passed as keyword arguments: 'b'
3.仅关键字参数 (3. Keyword only arguments)
To mark parameters as keyword-only, place an *
in the arguments list just before the first keyword-only parameter.
要将参数标记为仅关键字 ,请在参数列表中的第一个仅关键字参数之前放置*
。
def add(a,b,*,c,d):return a+b+c+d
print (add(3,4,c=1,d=2))#Output:10
If we specify positional arguments for keyword-only arguments it will raise TypeError.
如果我们为仅关键字参数指定位置参数,则会引发TypeError。
def add(a,b,*,c,d):return a+b+c+d
print (add(3,4,1,d=2))#Output:TypeError: add() takes 2 positional arguments but 3 positional arguments (and 1 keyword-only argument) were given
All 3 calling conventions used in the same function
同一功能中使用的所有3种调用约定
In the below-given example,function add
has all three arguments
在下面给出的示例中,function add
具有所有三个参数
a
,b
— positional only argumentsc
-positional or keyword argumentsd
-keyword-only arguments
a
, b
仅位置参数c
位置或关键字参数d
仅关键字参数
def add(a,b,/,c,*,d):return a+b+c+d
print (add(3,4,1,d=2))#Output:10
Important Points to remember:
要记住的要点:
-
Use positional-only if you want the name of the parameters to not be available to the user. This is useful when parameter names have no real meaning.
如果您不希望用户使用参数名称,请使用仅位置 。 当参数名称没有实际含义时,这很有用。
-
Use positional-only if you want to enforce the order of the arguments when the function is called.
如果要在调用函数时强制实参的顺序,请使用仅位置 。
-
Use keyword-only when names have meaning and the function definition is more understandable by being explicit with names.
仅当名称具有含义且通过使用名称明确表示功能定义时,才应使用仅关键字 。
-
Use keyword-only when you want to prevent users from relying on the position of the argument being passed.
当您要防止用户依赖传递的参数的位置时,请仅使用关键字 。
资源(Python文档): (Resources(Python Documentation):)
Defining functions
定义功能
default arguments
默认参数
keyword arguments
关键字参数
special parameters
特殊参数
arbitary argument list
任意论据表
翻译自: https://levelup.gitconnected.com/5-types-of-arguments-in-python-function-definition-e0e2a2cafd29
python函数参数定义