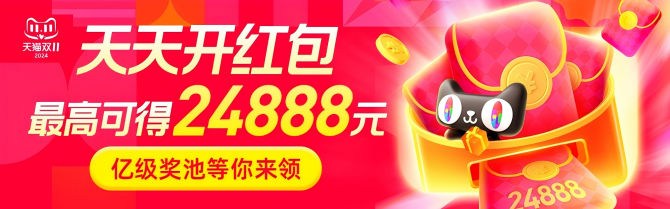
最近项目代码重构切换到了cmake的编译环境下。 有幸学习了一下cmake的一些基本的操作,记录一下。
0 . 创建一个工程
0.0 指明工程需要的cmake的最低版本(或者是最低到最高)
cmake_minimum_required(VERSION 3.1.2 FATAL_ERROR)
# cmake_minimum_required(VERSION <min>[...<policy_max>] [FATAL_ERROR])
0.1 指定工程的名称以及 编程语言等特点
project(myAwesomePrj)# project(<PROJECT-NAME> [<language-name>...])
# project(<PROJECT-NAME>
# [VERSION <major>[.<minor>[.<patch>[.<tweak>]]]]
# [DESCRIPTION <project-description-string>]
# [HOMEPAGE_URL <url-string>]
# [LANGUAGES <language-name>...])
1. cmake 生成工程
1.1 生成可执行文件
add_executable( application ${CMAKE_CURRENT_LIST_DIR}/1.cpp${CMAKE_CURRENT_LIST_DIR}/2.c
)
[jeason@centos7 cmake]$ mkdir build && cd build
[jeason@centos7 build]$ cmake ..
-- The C compiler identification is GNU 4.8.5
-- The CXX compiler identification is GNU 4.8.5
-- Check for working C compiler: /usr/bin/cc
-- Check for working C compiler: /usr/bin/cc -- works
-- Detecting C compiler ABI info
-- Detecting C compiler ABI info - done
-- Detecting C compile features
-- Detecting C compile features - done
-- Check for working CXX compiler: /usr/bin/c++
-- Check for working CXX compiler: /usr/bin/c++ -- works
-- Detecting CXX compiler ABI info
-- Detecting CXX compiler ABI info - done
-- Detecting CXX compile features
-- Detecting CXX compile features - done
-- Configuring done
-- Generating done
-- Build files have been written to: /home/jeason/cmake/build
[jeason@centos7 build]$ make
Scanning dependencies of target application
[ 33%] Building CXX object CMakeFiles/application.dir/1.cpp.o
[ 66%] Building C object CMakeFiles/application.dir/2.c.o
[100%] Linking CXX executable application
[100%] Built target application
[jeason@centos7 build]$ ls -l
total 40
-rwxrwxr-x. 1 jeason jeason 8376 Dec 5 09:19 application
-rw-rw-r--. 1 jeason jeason 11635 Dec 5 09:19 CMakeCache.txt
drwxrwxr-x. 5 jeason jeason 4096 Dec 5 09:19 CMakeFiles
-rw-rw-r--. 1 jeason jeason 1353 Dec 5 09:19 cmake_install.cmake
-rw-rw-r--. 1 jeason jeason 5278 Dec 5 09:19 Makefile
[jeason@centos7 build]$
1.2 生成静态库
cmake_minimum_required(VERSION 3.1.6)
project(myAwesomePrj)add_library( temp STATIC${CMAKE_CURRENT_LIST_DIR}/1.cpp${CMAKE_CURRENT_LIST_DIR}/2.c
)
[jeason@centos7 cmake]$ mkdir build && cd build
[jeason@centos7 build]$ cmake ..
-- The C compiler identification is GNU 4.8.5
-- The CXX compiler identification is GNU 4.8.5
-- Check for working C compiler: /usr/bin/cc
-- Check for working C compiler: /usr/bin/cc -- works
-- Detecting C compiler ABI info
-- Detecting C compiler ABI info - done
-- Detecting C compile features
-- Detecting C compile features - done
-- Check for working CXX compiler: /usr/bin/c++
-- Check for working CXX compiler: /usr/bin/c++ -- works
-- Detecting CXX compiler ABI info
-- Detecting CXX compiler ABI info - done
-- Detecting CXX compile features
-- Detecting CXX compile features - done
-- Configuring done
-- Generating done
-- Build files have been written to: /home/jeason/cmake/build
[jeason@centos7 build]$ make
Scanning dependencies of target temp
[ 33%] Building CXX object CMakeFiles/temp.dir/1.cpp.o
[ 66%] Building C object CMakeFiles/temp.dir/2.c.o
[100%] Linking CXX static library libtemp.a
[100%] Built target temp
[jeason@centos7 build]$ ls -l
total 32
-rw-rw-r--. 1 jeason jeason 11687 Dec 5 09:27 CMakeCache.txt
drwxrwxr-x. 5 jeason jeason 4096 Dec 5 09:27 CMakeFiles
-rw-rw-r--. 1 jeason jeason 1353 Dec 5 09:27 cmake_install.cmake
-rw-rw-r--. 1 jeason jeason 2370 Dec 5 09:27 libtemp.a
-rw-rw-r--. 1 jeason jeason 5131 Dec 5 09:27 Makefile
[jeason@centos7 build]$
1.3 生成动态库
cmake_minimum_required(VERSION 3.1.6)
project(myAwesomePrj)add_library( temp SHARED${CMAKE_CURRENT_LIST_DIR}/1.cpp${CMAKE_CURRENT_LIST_DIR}/2.c
)
[jeason@centos7 cmake]$ mkdir build && cd build
[jeason@centos7 build]$ cmake ..
-- The C compiler identification is GNU 4.8.5
-- The CXX compiler identification is GNU 4.8.5
-- Check for working C compiler: /usr/bin/cc
-- Check for working C compiler: /usr/bin/cc -- works
-- Detecting C compiler ABI info
-- Detecting C compiler ABI info - done
-- Detecting C compile features
-- Detecting C compile features - done
-- Check for working CXX compiler: /usr/bin/c++
-- Check for working CXX compiler: /usr/bin/c++ -- works
-- Detecting CXX compiler ABI info
-- Detecting CXX compiler ABI info - done
-- Detecting CXX compile features
-- Detecting CXX compile features - done
-- Configuring done
-- Generating done
-- Build files have been written to: /home/jeason/cmake/build
[jeason@centos7 build]$ make
Scanning dependencies of target temp
[ 33%] Building CXX object CMakeFiles/temp.dir/1.cpp.o
[ 66%] Building C object CMakeFiles/temp.dir/2.c.o
[100%] Linking CXX shared library libtemp.so
[100%] Built target temp
[jeason@centos7 build]$ ls -l
total 36
-rw-rw-r--. 1 jeason jeason 11687 Dec 5 09:29 CMakeCache.txt
drwxrwxr-x. 5 jeason jeason 4096 Dec 5 09:29 CMakeFiles
-rw-rw-r--. 1 jeason jeason 1353 Dec 5 09:29 cmake_install.cmake
-rwxrwxr-x. 1 jeason jeason 7912 Dec 5 09:29 libtemp.so
-rw-rw-r--. 1 jeason jeason 5131 Dec 5 09:29 Makefile
[jeason@centos7 build]$
file(GLOB_RECURSE some_prj_src "*${CMAKE_CURRENT_LIST_DIR}.cpp")
file(GLOB_RECURSE some_prj_src "*.cpp")
2. 一些辅助工具
2.1 打印提示信息
cmake_minimum_required(VERSION 3.1.6)
project(myAwesomePrj)
message("hello world ..... ")
[jeason@centos7 build]$ cmake ..
hello world .....
-- Configuring done
-- Generating done
-- Build files have been written to: /home/jeason/cmake/build
2. 辅助查找文件
cmake_minimum_required(VERSION 3.1.6)
project(myAwesomePrj)
file(GLOB_RECURSE file_out_list_c "*.c")
file(GLOB_RECURSE file_out_list_cpp "*.cpp")
file(GLOB_RECURSE file_out_list_c_cpp "*.cpp" "*.c")
message("file find file_out_list_c = ${file_out_list_c}")
message("file find file_out_list_cpp = ${file_out_list_cpp}")
message("file find file_out_list_c_cpp = ${file_out_list_c_cpp}") message("----------------------------------------------------------------------------") foreach(files ${file_out_list_c})message("----- ${files}")
endforeach()
[jeason@centos7 build]$ cmake ..
file find file_out_list_c = /home/jeason/cmake/2.c;/home/jeason/cmake/build/CMakeFiles/3.6.2/CompilerIdC/CMakeCCompilerId.c;/home/jeason/cmake/build/CMakeFiles/feature_tests.c
file find file_out_list_cpp = /home/jeason/cmake/1.cpp;/home/jeason/cmake/build/CMakeFiles/3.6.2/CompilerIdCXX/CMakeCXXCompilerId.cpp
file find file_out_list_c_cpp = /home/jeason/cmake/1.cpp;/home/jeason/cmake/build/CMakeFiles/3.6.2/CompilerIdCXX/CMakeCXXCompilerId.cpp;/home/jeason/cmake/2.c;/home/jeason/cmake/build/CMakeFiles/3.6.2/CompilerIdC/CMakeCCompilerId.c;/home/jeason/cmake/build/CMakeFiles/feature_tests.c
----------------------------------------------------------------------------
----- /home/jeason/cmake/2.c
----- /home/jeason/cmake/build/CMakeFiles/3.6.2/CompilerIdC/CMakeCCompilerId.c
----- /home/jeason/cmake/build/CMakeFiles/feature_tests.c
-- Configuring done
-- Generating done
-- Build files have been written to: /home/jeason/cmake/build
[jeason@centos7 build]$
还有亿点点细节没写完 有点想不起来了,明天看看代码再做笔记!!!
cmake 是非常强大的! 语法也是清晰易懂的