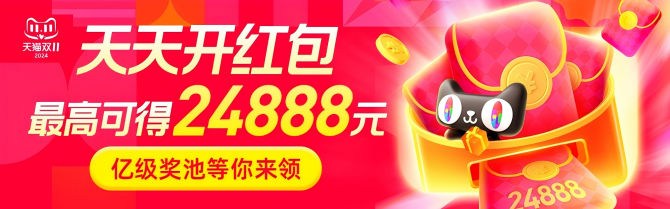
泰克-吉时利-2200-远程操作
前言
以下是调试泰克吉时利2200直流电源远程操作的调试经验,有需要的可以参考。
一、VISA驱动安装
Visa驱动在NI官网下载,如下地址:
[https://www.ni.com/zh-cn/support/downloads/drivers/download.ni-visa.html#346210]
二、电流计设置
Shift–>Menu–>Communication–>USBTMC
三、Visa协议头文件,静态库
visa.h,visatype.h
64位Windows:
C:\Program Files (x86)\IVI Foundation\VISA\WinNT\include
C:\Program Files\IVI Foundation\VISA\Win64\include
32位Windows:
C:\Program Files\IVI Foundation\VISA\WinNT\include
visa32/64.lib
C:\Program Files\IVI Foundation\VISA\Win64\Lib_x64\msc
链接器–>常规–>附加目录库–>添加静态库地址(C:\Program Files\IVI Foundation\VISA\Win64\Lib_x64\msc)
链接器–>输入–>附加依赖库–>添加静态库名(visa64.lib/visa64.lib)
四、实现代码
//代码截取吉时利2200 spec
#define _CRT_SECURE_NO_WARNINGS
#include <C:\\Program Files\\IVI Foundation\\VISA\\Win64\\Include\\visa.h>
#include <C:\\Program Files\\IVI Foundation\\VISA\\Win64\\Include\\visatype.h>
#include <stdarg.h>
#include <stdio.h>
#include <string.h>
#include <time.h>
#include <conio.h>
#include <stdlib.h>ViSession defaultRM; //Resource manager id
ViSession PWS2200; //Identifies the power supplylong ErrorStatus;
char commandString[256];
char ReadBuffer[256];
void OpenPort();
void SendSCPI(char* pString);
void CheckError(char* pMessage);
void delay(clock_t wait);
void ClosePort();int main()
{double voltage;char Buffer[256];double current;OpenPort();//Query the power supply id, read response and print itsprintf(Buffer, "*IDN?");SendSCPI(Buffer);printf("Instrument identification string:%s \n", Buffer);SendSCPI("*RST"); //reset the power supplySendSCPI("CURRENT 0.1A"); //set the current to 0.1ASendSCPI("VOLTAGE 3V"); //set the voltage to 3VSendSCPI("OUTPUT 1"); // turn output onvoltage = 5.0;current = 0.2;printf("Setting voltage(V) & current(A): %f,%f \n",voltage, current);ErrorStatus = viPrintf(PWS2200, "VOLT %f\n", voltage); //set the output voltageCheckError("Unable to set voltage");ErrorStatus = viPrintf(PWS2200, "CURRENT %f\n", current);//set the output currentCheckError("Unable to set current");ErrorStatus = viPrintf(PWS2200, "MEASURE:VOLTAGE?\n");//measure the output voltageCheckError("Unable to write the device");ErrorStatus = viScanf(PWS2200, "%f", &voltage); //retrieve readingCheckError("Unable to read voltage");ErrorStatus = viPrintf(PWS2200, "MEASURE:CURRENT?\n");//measure the output currentCheckError("Unable to write the device");ErrorStatus = viScanf(PWS2200, "%f", ¤t); //retrieve readingCheckError("Unable to read current");printf("Measured voltage(V) & current(A): %f,%f \n",voltage, current);SendSCPI("OUTPUT 0"); //turn output offClosePort();while (1);//return 0;
}
void OpenPort()
{//Open communication session with the power supply, and put the power supply in remoteErrorStatus = viOpenDefaultRM(&defaultRM);ErrorStatus = viOpen(defaultRM,"USB0::0x05E6::0x2200::9203766::INSTR", 0, 0, &PWS2200);CheckError("Unable to open the port");SendSCPI("SYSTEM:REMOTE");
}
void SendSCPI(char* pString)
{char* pdest;strcpy(commandString, pString);strcat(commandString, "\n");ErrorStatus = viPrintf(PWS2200, commandString);CheckError("Can't Write to Power Supply");pdest = strchr(commandString, '?'); //Search for query commandif (pdest != NULL){ErrorStatus = viBufRead(PWS2200, (ViBuf)ReadBuffer,sizeof(ReadBuffer), VI_NULL);CheckError("Can't read from driver");strcpy(pString, ReadBuffer);}
}
void ClosePort()
{viClose(PWS2200);viClose(defaultRM);
}
void CheckError(char* pMessage)
{if (ErrorStatus != VI_SUCCESS){printf("\n %s", pMessage);ClosePort();exit(0);}
}
void delay(clock_t wait)
{clock_t goal;goal = wait + clock();while (goal > clock());
}